ในบางครั้งการเขียน app เพื่อ access location ปัจจุบันของผู้ใช้ หรือต้องการสั่งให้โทรศัพท์สั่นตาม activity ที่ตั้งไว้ หรือแม้แต่จะ access ข้อมูล device information ของอุปกรณ์ ไม่ใช่เรื่องง่ายเลยสำหรับเหล่า developer ที่เขียน mobile app ยิ่งมีหลาย platform ไม่ว่าจะเป็น android , iOS, Windows ก็มีวิธีการเขียน code การขอ Access permission คนละรูปแบบไปอีก ซึ่งบทความนี้ผู้เขียนจะมาแนะนำให้รู้จักกับ Xamarin Essentials ที่จะมาเป็นตัวช่วยให้ผู้พัฒนาเขียน code แก้ปัญหาเหล่านี้ได้ง่ายขึ้นนั่นเอง
Xamarin.Essentials คืออะไร?
คือ official library ที่ทาง Microsoft ได้พัฒนาและรวบรวม library ที่เป็น cross-platform APIs สำหรับให้นักพัฒนา mobile app ที่พัฒนาด้วย Xamarin.Forms ได้ใช้งานในการเข้าถึง native features ของแต่ละ platforms ด้วยการเขียน code จากที่เดียว
มีอะไรให้เรียกใช้บ้าง
ปัจจุบันมีทั้งหมด 33 feature ให้เรียกใช้งาน อ้างอิงจาก Microsoft Doc ซึ่งมีดังนี้
- Accelerometer – Retrieve acceleration data of the device in three dimensional space.
- App Information – Find out information about the application.
- Barometer – Monitor the barometer for pressure changes.
- Battery – Easily detect battery level, source, and state.
- Clipboard – Quickly and easily set or read text on the clipboard.
- Color Converters – Helper methods for System.Drawing.Color.
- Compass – Monitor compass for changes.
- Connectivity – Check connectivity state and detect changes.
- Detect Shake – Detect a shake movement of the device.
- Device Display Information – Get the device’s screen metrics and orientation.
- Device Information – Find out about the device with ease.
- Email – Easily send email messages.
- File System Helpers – Easily save files to app data.
- Flashlight – A simple way to turn the flashlight on/off.
- Geocoding – Geocode and reverse geocode addresses and coordinates.
- Geolocation – Retrieve the device’s GPS location.
- Gyroscope – Track rotation around the device’s three primary axes.
- Launcher – Enables an application to open a URI by the system.
- Magnetometer – Detect device’s orientation relative to Earth’s magnetic field.
- MainThread – Run code on the application’s main thread.
- Maps – Open the maps application to a specific location.
- Open Browser – Quickly and easily open a browser to a specific website.
- Orientation Sensor – Retrieve the orientation of the device in three dimensional space.
- Phone Dialer – Open the phone dialer.
- Platform Extensions – Helper methods for converting Rect, Size, and Point.
- Preferences – Quickly and easily add persistent preferences.
- Secure Storage – Securely store data.
- Share – Send text and website uris to other apps.
- SMS – Create an SMS message for sending.
- Text-to-Speech – Vocalize text on the device.
- Unit Converters – Helper methods to convert units.
- Version Tracking – Track the applications version and build numbers.
- Vibrate – Make the device vibrate.
วิธีใช้งาน
เปิด Xamarin.Forms project ขึ้นมา และไปที่เมนู Tools -> Nuget Package Manager -> Manage Nuget Package for Solution และในช่อง Search ให้ค้นหา Xamarin.Essentials ดังรูป
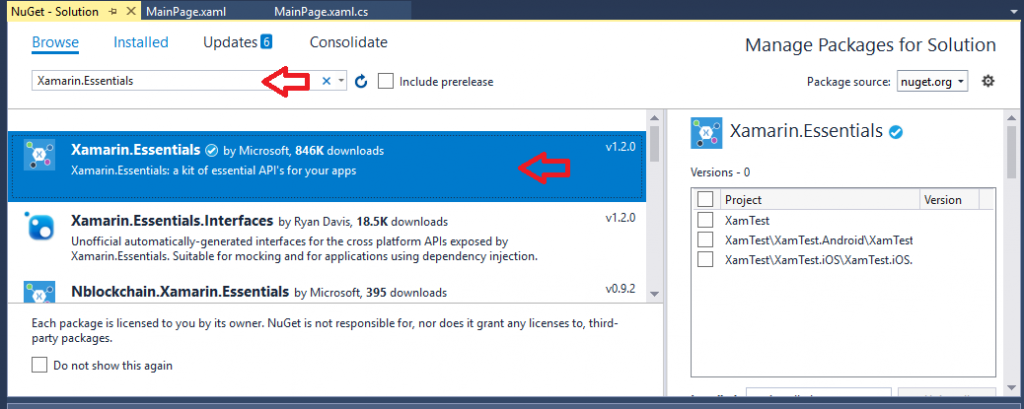
ที่ฝั่งขวา ให้เราเลือก install ลงทุก project ทั้งในส่วน shared project ตรงกลาง และ specific platform project (android และ ios) แล้วกดปุ่ม Install ดังรูป
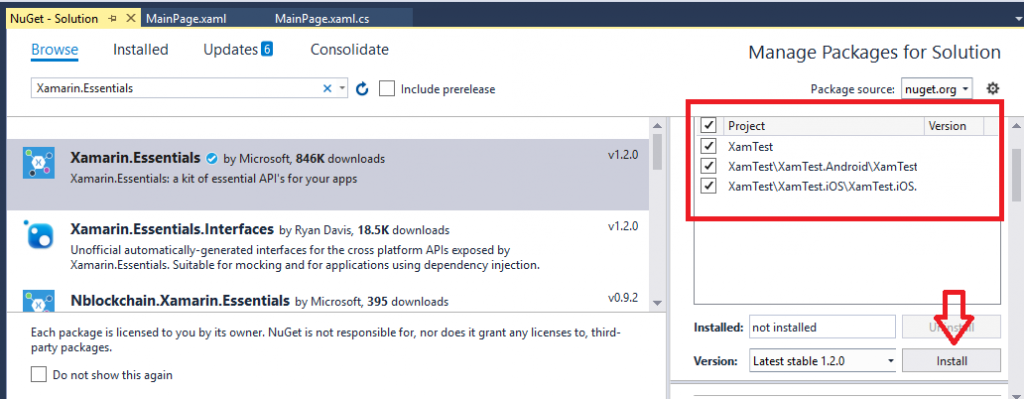
ถึงตอนนี้ Xamarin.Essentials ถูกเพิ่มเข้าไปใน Reference package ของแต่ละ project เรียบร้อย แต่เรายังต้อง Config ค่าเพิ่มเติมนิดหน่อยเฉพาะ android project เท่านั้น (ios project ไม่ต้องทำอะไรก็ใช้งานได้เลย) เพื่อให้ Xamarin.Essential สามารถทำงานได้ โดยทำการเพิ่มเติม code ที่ MainActivity.cs เพื่อ initial Xamarin.Essentials ใน OnCreate() และทำการ override OnrequestPermissionResult() ดัง code ข้างล่าง เป็นอันเสร็จเรียบร้อยพร้อมให้ให้เขียน code เรียกใช้งาน feature ต่างๆ ได้เลยค่ะ
protected override void OnCreate(Bundle bundle) { ... global::Xamarin.Forms.Forms.Init(this, bundle); Xamarin.Essentials.Platform.Init(this, bundle); ... } public override void OnRequestPermissionsResult(int requestCode, string[] permissions, [GeneratedEnum] Android.Content.PM.Permission[] grantResults) { Xamarin.Essentials.Platform.OnRequestPermissionsResult(requestCode, permissions, grantResults); base.OnRequestPermissionsResult(requestCode, permissions, grantResults); }
ตัวอย่าง
ให้ทำการ using Xamarin.Essentials ที่ class ที่เราจะเรียกใช้งาน หลังจากนั้นสามารถเขียน code ใช้งาน method ต่างๆได้เลยค่ะ ขอยกตัวอย่างบาง feature ดังต่อไปนี้นะคะ
1. ส่ง SMS
หากเราต้องการส่ง SMS จาก Xamarin.Forms app ของเรา ก็เขียน code ดังตัวอย่างข้างล่างนี้
public class SmsTest { public async Task SendSms(string messageText, string recipient) { try { var message = new SmsMessage(messageText, new []{ recipient }); await Sms.ComposeAsync(message); } catch (FeatureNotSupportedException ex) { // Sms is not supported on this device. } catch (Exception ex) { // Other error has occurred. } } }
2. Get Location
อยากรู้ตำแหน่ง Location ล่าสุดของ device ก็เขียน code ดังนี้
try { var location = await Geolocation.GetLastKnownLocationAsync(); if (location != null) { Console.WriteLine($"Latitude: {location.Latitude}, Longitude: {location.Longitude}, Altitude: {location.Altitude}"); } } catch (FeatureNotSupportedException fnsEx) { // Handle not supported on device exception }
ผู้เขียนขอยกตัวอย่างเพียงเท่านี้ ส่วนการเรียกใช้งาน feature อื่น สามารถอ่านได้จาก doc ของทาง Microsoft Xamarin.Essentials ได้เลยค่ะ ไม่ยากเลยใช่มั้ยคะ เขียน code เพียงไม่กี่บรรทัด เราก็สามารถเข้าถึง native feature ของแต่ละ platform ได้แล้วว ^^